This can be intimidating:
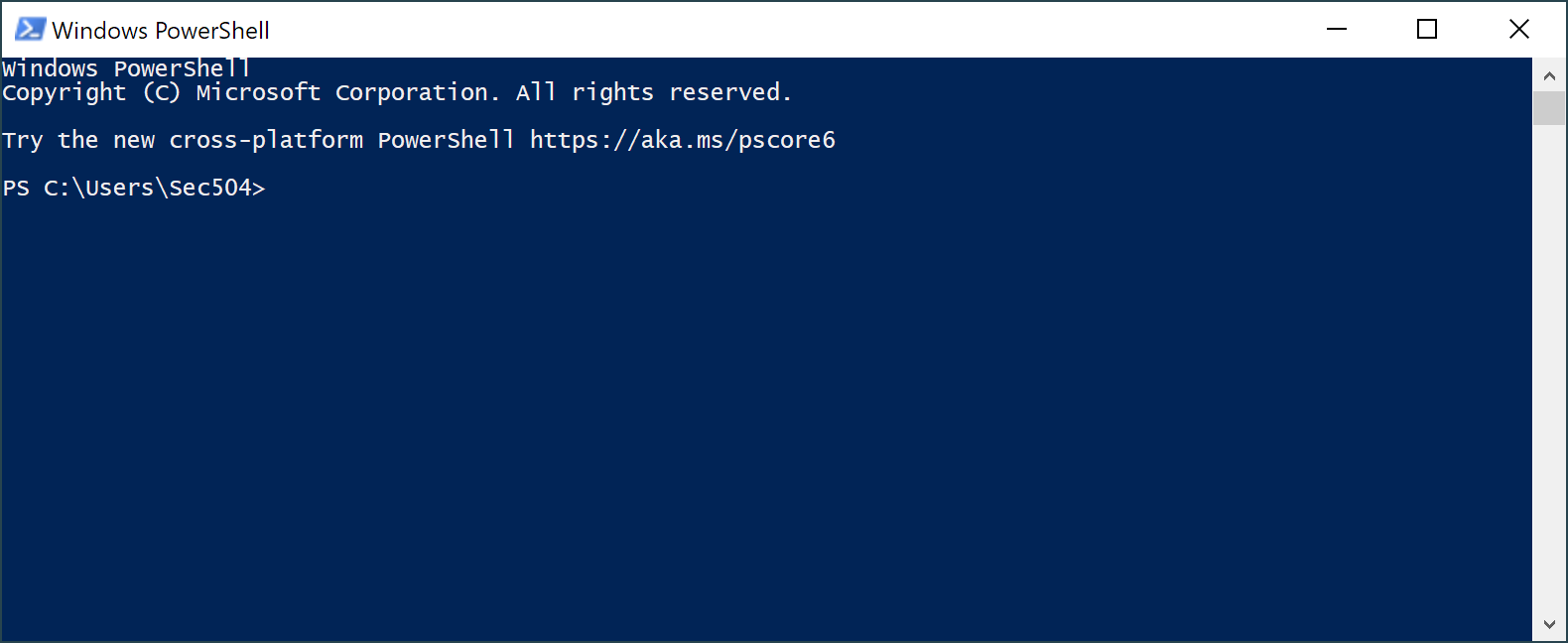
PowerShell is powerful, and there's amazing things that we can do with it, but for a long time, I couldn't get past the how do I start phase of learning PowerShell. In this article I'll talk about the 5 tips that helped me get started with PowerShell.
I encourage you to follow along as you read this article. Open PowerShell on Windows and enter the commands in the PowerShell examples.
Verb-Noun
PowerShell commands are build on a convention: Verb-Noun. You've probably seen this a bunch of times before in commands like Get-ChildItem, Get-Date, Stop-Process, Write-Host, etc. While not everything in PowerShell follows this convention, most commands do, and it helps to predict and anticipate what the right command would be for a certain task.
Let's explore this. Start PowerShell (Windows, Linux or macOS is fine) and enter the Get-Verb command:
PS C:\Users\Sec504> Get-Verb
Verb Group
---- -----
Add Common
Clear Common
Close Common
Copy Common
Enter Common
...lots of verbs removed for brevity
Revoke Security
Unblock Security
Unprotect Security
Use Other
PS C:\Users\Sec504>
PowerShell commands use the verb to describe the general scope of the action; that is, the verb tells you what the command is going to do, broadly.
Verb | Action | Example |
---|---|---|
Copy | Copies a resource to a new name | Copy-Item |
Get | Retrieves something | Get-Content |
Set | Sets something | Set-AppLockerPolicy |
Out | Sends data out of the PowerShell session | Out-File |
New | Creates a resource | New-FileShare |
From your PowerShell session, run the Get-Noun command:
PS C:\Users\Sec504> Get-Noun
Get-Noun : The term 'Get-Noun' is not recognized as the name of a cmdlet, function, script file, or operable
program. Check the spelling of the name, or if a path was included, verify that the path is correct and try
again.
Sorry, that was a trick. There is no Get-Noun command; PowerShell verbs are few, but nouns are many. Fortunately, there are commands to help us figure out which Verb-Noun combination we need to accomplish a task.
Get-Command
From your PowerShell session, run the Get-Command, umm, command:
PS C:\Users\Sec504> Get-Command
CommandType Name Version Source
----------- ---- ------- ------
Alias Add-AppPackage 2.0.1.0 Appx
Alias Add-AppPackageVolume 2.0.1.0 Appx
Alias Add-AppProvisionedPackage 3.0 Dism
Alias Add-ProvisionedAppPackage 3.0 Dism
Alias Add-ProvisionedAppxPackage 3.0 Dism
...
Cmdlet Write-Progress 3.1.0.0 Microsoft.PowerShell.Utility
Cmdlet Write-Verbose 3.1.0.0 Microsoft.PowerShell.Utility
Cmdlet Write-Warning 3.1.0.0 Microsoft.PowerShell.Utility
Get-Command returns all of the PowerShell commands available (functions, scripts, cmdlets, and more). Run by itself, it's a lot of output and it doesn't provide any immediate insight into what command do I use to solve this problem, but you can also specify a partial string to match as an argument. For example, let's say you want to restart a Windows service:
PS C:\Users\Sec504> Get-Command *service*
CommandType Name Version Source
----------- ---- ------- ------
Function Get-NetFirewallServiceFilter 2.0.0.0 NetSecurity
Function Set-NetFirewallServiceFilter 2.0.0.0 NetSecurity
Cmdlet Get-Service 3.1.0.0 Microsoft.PowerShell.Management
Cmdlet New-Service 3.1.0.0 Microsoft.PowerShell.Management
Cmdlet New-WebServiceProxy 3.1.0.0 Microsoft.PowerShell.Management
Cmdlet Restart-Service 3.1.0.0 Microsoft.PowerShell.Management
Cmdlet Resume-Service 3.1.0.0 Microsoft.PowerShell.Management
Cmdlet Set-Service 3.1.0.0 Microsoft.PowerShell.Management
Cmdlet Start-Service 3.1.0.0 Microsoft.PowerShell.Management
Cmdlet Stop-Service 3.1.0.0 Microsoft.PowerShell.Management
Cmdlet Suspend-Service 3.1.0.0 Microsoft.PowerShell.Management
Application AgentService.exe 10.0.19... C:\WINDOWS\system32\AgentService.exe
Application SecurityHealthService.exe 4.18.19... C:\WINDOWS\system32\SecurityHealthService.exe
Application SensorDataService.exe 10.0.19... C:\WINDOWS\system32\SensorDataService.exe
Application services.exe 10.0.19... C:\WINDOWS\system32\services.exe
Application services.msc 0.0.0.0 C:\WINDOWS\system32\services.msc
Application TieringEngineService.exe 10.0.19... C:\WINDOWS\system32\TieringEngineService.exe
Application vm3dservice.exe 0.0.0.0 C:\WINDOWS\system32\vm3dservice.exe
Application Windows.WARP.JITService.exe 0.0.0.0 C:\WINDOWS\system32\Windows.WARP.JITService.exe
Using the * wildcard, you can specify a partial string to match. Get-Command is very helpful, allowing us to search for commands that match a specific keyword.
This is a really useful feature of PowerShell, but it doesn't always help. For example, let's say you want to delete a file using PowerShell:
PS C:\Users\Sec504> Get-Command *File*
CommandType Name Version Source
----------- ---- ------- ------
Alias Set-AppPackageProvisionedDataFile 3.0 Dism
Alias Set-ProvisionedAppPackageDataFile 3.0 Dism
Alias Set-ProvisionedAppXDataFile 3.0 Dism
Alias Write-FileSystemCache 2.0.0.0 Storage
Function Block-FileShareAccess 2.0.0.0 Storage
Function Clear-FileStorageTier 2.0.0.0 Storage
Function Close-SmbOpenFile 2.0.0.0 SmbShare
...
Application FileHistory.exe 10.0.19... C:\WINDOWS\system32\FileHistory.exe
Application forfiles.exe 10.0.19... C:\WINDOWS\system32\forfiles.exe
Application openfiles.exe 10.0.19... C:\WINDOWS\system32\openfiles.exe
You may expect that this would be Remove-File, but that command doesn't exist. PowerShell uses item as the noun to represent files and other objects. This can be tricky at first, but after you start working with commands like Get-Item and Get-ChildItem it becomes more intuitive.
Tab Completion
In PowerShell, you can type a partial command name, then press the Tab key to complete it. You can also do this for file and directory names, registry keys, and other items. You can also do this for parameters to PowerShell commands as well.
If you remember the beginning of the command, type in a few characters and press Tab. PowerShell will show you the first name that matches (alphabetically), but you can keep pressing Tab to cycle through all available options. You can also do this for PowerShell command parameters, file names, and more!
Make Tab completion a regular part of every PowerShell session.
Get-Help
Reading documentation is a essential part of skill development, and PowerShell isn't different. You're going to have to read the fine manual, at least when you are getting started, and then only when you want to look something up later.
PowerShell has integrated help that is regularly maintained and updated. From your PowerShell session, run Get-Help:
PS C:\Users\Sec504> Get-Help
TOPIC
Windows PowerShell Help System
SHORT DESCRIPTION
Displays help about Windows PowerShell cmdlets and concepts.
LONG DESCRIPTION
Windows PowerShell Help describes Windows PowerShell cmdlets,
functions, scripts, and modules, and explains concepts, including
the elements of the Windows PowerShell language.
...
The Get-Help command will be your guide to understanding the parameters and options for PowerShell commands. Let's check out the help for Get-EventLog:
PS C:\Users\Sec504> Get-Help Get-EventLog
NAME
Get-EventLog
SYNOPSIS
Gets the events in an event log, or a list of the event logs, on the local computer or
remote computers.
SYNTAX
Get-EventLog [-LogName] <System.String> [[-InstanceId] <System.Int64[]>] [-After
<System.DateTime>] [-AsBaseObject] [-Before <System.DateTime>] [-ComputerName
<System.String[]>] [-EntryType {Error | Information | FailureAudit | SuccessAudit |
Warning}] [-Index <System.Int32[]>] [-Message <System.String>] [-Newest <System.Int32>]
[-Source <System.String[]>] [-UserName <System.String[]>] [<CommonParameters>]
...
You used tab completion for Get-Help and Get-EventLog, right?
The help information can be overwhelming at first, but there are some rules to the formatting that help:
- Square brackets with a name in the middle (e.g, [-LogName]) indicates that the parameter is optional
- Angle brackets indicate that a value is needed using the datatype specified (e.g. <System.String> indicates that you need to supply a string value
- The combined [-LogName] <System.String> indicates that you can specify -LogName or not (since it's optional) but you must specify the string (in this case, the name of the event log)
- Empty square brackets after a data type (e.g., <System.Int64[]>) indicates that the parameter accepts more than one value (more than one integer, in this example)
- Curly braces {} indicate a collection of choices separated by |; here, [-EntryType {Error | Information | FailureAudit | SuccessAudit | Warning}] indicates that the EntryType parameter is optional (since it's in [...] brackets), and the only allowed arguments are Error, Information, FailureAudit, SuccessAudit, or Warning
Tip: More information on reading help documentation from Microsoft is available in the Microsoft PowerShell documentation Appendix A - Help Syntax
Still, it's easy to get lost reading the help syntax. Fortunately, Microsoft makes examples available. Run the previous PowerShell command again, adding -Examples:
PS C:\Users\Sec504> Get-Help Get-EventLog -Examples
NAME
Get-EventLog
SYNOPSIS
Gets the events in an event log, or a list of the event logs, on the local computer or
remote computers.
------- Example 1: Get event logs on the local computer -------
Get-EventLog -List
Max(K) Retain OverflowAction Entries Log
------ ------ -------------- ------- ---
15,168 0 OverwriteAsNeeded 20,792 Application
15,168 0 OverwriteAsNeeded 12,559 System
15,360 0 OverwriteAsNeeded 11,173 Windows PowerShell
The `Get-EventLog` cmdlet uses the List parameter to display the available logs.
Example 2: Get recent entries from an event log on the local computer
Get-EventLog -LogName System -Newest 5
...
Scrolling up and down in your terminal isn't fun, do PowerShell also offers the -ShowWindow argument. Now you can get the help and the PowerShell session side-by-side:
You can also run update-help to get the current help information (but you must do this from an Administrative PowerShell session).
Aliases
PowerShell commands can be long and awkward to type. Even with tab completion, a simple command can take up multiple lines in your terminal. Aliases are shortcuts, typically just a few characters to enter a command. Aliases are less intuitive, but they can be faster when you're trying to get something done.
From your PowerShell session, run Get-Alias to see a list of aliases:
PS C:\Users\Sec504> Get-Alias
CommandType Name Version Source
----------- ---- ------- ------
Alias % -> ForEach-Object
Alias ? -> Where-Object
Alias ac -> Add-Content
Alias asnp -> Add-PSSnapin
Alias cat -> Get-Content
Alias cd -> Set-Location
Alias CFS -> ConvertFrom-String 3.1.0.0 Microsoft.PowerShell.Utility
Alias chdir -> Set-Location
Alias clc -> Clear-Content
...
Take a look at cd. If you've used Windows CMD, macOS terminal, or Linux shells, you've probably seen this command before. In PowerShell, cd is an alias for Set-Location. Both commands (the cd alias or the Set-Location cmdlet) will accomplish the same thing. Use whatever is convenient for you!
If you want to look up a specific alias to find out what it does, you can use Get-Alias <COMMAND>. Try it for the wget alias:
PS C:\Users\Sec504> Get-Alias wget
CommandType Name Version Source
----------- ---- ------- ------
Alias wget -> Invoke-WebRequest
PowerShell will run the Invoke-WebRequest cmdlet anytime you run the wget command. To look up the alias for a PowerShell command, you can use Get-Alias with the -Definition argument. Try it for the Get-Location cmdlet:
PS C:\Users\Sec504> Get-Alias -Definition Get-Location
CommandType Name Version Source
----------- ---- ------- ------
Alias gl -> Get-Location
Alias pwd -> Get-Location
Here we see two options: both the gl and the pwd aliases will run the Get-Location cmdlet.
Note: You can also look at the help information for a command to identify the default aliases.
Summary
To recap, here's the 5 tips for getting started with PowerShell:
- Remember Verb-Noun is the format for PowerShell commands. You can use Get-Verb to see a list of supported verbs.
- Get-Command will show you a list of all the available commands in PowerShell. Specify a partial string with wildcards for a quick command lookup.
- Tab completion will help you navigate PowerShell commands, parameters, file names, and more. Make it a regular part of your PowerShell use.
- Get-Help is actually useful documentation.
- Use the alias in PowerShell for the commands you are already familiar with from other scripting and shell environments. Use Get-Alias to look up the alias names.
Put these tips into practice and you'll be well on your way to making PowerShell intimidation a thing of the past!
-Joshua Wright
Jump to another Month of PowerShell article here:
- Embracing the Pipeline
- Working with the Registry
- Abusing Get-Clipboard
- Getting Object Properties for Windows Service Dependencies
- Profile Hack for Easy Base64 Encoding and Decoding
- The Power of $PROFILE
- String Substitution
- Threat Hunting with PowerShell Differential Analysis
- Windows File Server Enumeration
- Working with the Event Log, Part 1
- Working with the Event Log, Part 2: Threat Hunting with Event Logs
- Working with Log Files
- Working with the Event Log, Part 3 - Accessing Message Elements
- Merging Two Files (Understanding ForEach)
- Working with the Event Log, Part 4 - Tweaking Event Log Settings
- Solving Problems DeepBlueCLI, Syslog, and JSON)
- Using The Grouping Operator (a.k.a. What are all these ()?)
- Working with Kilobytes, Megabytes, and Gigabytes
- Recording Your Session with Start-Transcript
- Process Threat Hunting, Part 1
- The Curious Case of AD User Properties
- Process Threat Hunting, Part 2
- PowerShell Version of Keeper (Save Useful Command Lines)
- PowerShell Remoting, Part 1
- PowerShell Fileless Malware with Get-Clipboard
- Renaming Groups of Files
- PowerShell Remoting, Part 2
- Keyboard Shortcuts Like a Boss
Joshua Wright is the author of SANS SEC504: Hacker Tools, Techniques, and Incident Handling, a faculty fellow for the SANS Institute, and a senior technical director at Counter Hack.