Tags:
This is a guest post from security researcher Nitesh Dhanjani. Nitesh will be giving a talk on "Hacking and Securing Next Generation iPhone and iPad Apps" at SANS AppSec 2011.
Millions of iOS users and developers have come to rely on Apple's Push Notification Service (APN). In this article, I will briefly introduce details of how APN works and present scenarios of how insecure implementations can be abused by malicious parties.
Apple's iOS allows some tasks to truly execute in the background when a user switches to another app (or goes back to the home screen), yet most apps will return and resume from a frozen state right where they left off. Apple's implementation helps preserve battery life by providing the user the illusion that iOS allows for full-fledged multi-tasking between 3rd party apps.
This setup makes it hard for apps to implement features that rely on full-fledged multi-tasking. For example, an Instant Messaging app will want to alert the user of a new incoming message even if the user is using another app. To enable this functionality, the APN service can be used by app vendors to push notifications to the user's device even when the app is in a frozen state (i.e. the user is using another app or is on the home screen).
.png)
There are 3 types of push notifications in iOS: alerts, badges, and sounds. Figure 1 shows a screen-shot illustrating both an alert and a badge notification.
iOS devices maintain a persistent connection to the APN servers. Providers, i.e. 3rd party app vendors, must connect to the APN to route the notification to a target device.
Implementing Push Notifications
So how can an app developer implement push notifications? For explicit details, I'd recommend going through Apple's excellent Local and Push Notifications Programming Guide. However, I will briefly cover the steps below with emphasis on steps that have interesting security implications and tie them to abuse cases and recommendations.
1. Create an App ID on Apple's iOS Provisioning Portal
This step requires you sign up for a developer account ($99). This is straight-forward: just click on App IDs in the provisioning portal and then select New App ID. Next, enter text in the Description field that describes your app. The Bundle Seed ID is used to signify if you want multiple apps to share the same Keychain store.
The Bundle Identifier is where it gets interesting: you need to enter a unique identifier for your App ID. Apple recommends using a reverse-domain name style string for this.
.png)
The Bundle Identifier is significant from a security perspective because it makes its way into the provider certificate we will create later (also referred to as the topic). The APN trusts this field in the certificate to figure out which app in the target user's device to convey the push message to. As Figure 2 illustrates, an attempt to create an App ID with the Bundle Identifier of com.facebook.facebook is promptly refused as a duplicate, i.e. the popular Facebook iOS app is probably using this in it's certificate. Therefore, if a malicious entity were to bypass the provisioning portal's restrictions against allowing an existing Bundle Identifier, then he or she could possibly influence Apple to provision a certificate that can be abused to send push notifications to users of another app (but the malicious user would still need to know the target user's device-token discussed later in this article).
2. Create a Certificate Signing Request (CSR) to have Apple generate an APN certificate
In this step, Keychain on the desktop is used to generate a public and private cryptographic key pair. The private key stays on the desktop. The public key is included in the CSR and uploaded to Apple. The APN certificate that Apple provides back is specific to the app and tied to a specific App ID generated in step 1.
3. Create a Provisioning Profile to deploy your App into a test iOS device with push notifications enabled for the App ID you selected
This step is pretty straight forward and described in Apple's documentation linked above.
4. Export the APN certificate to the server side
As described in Apple's documentation, you can choose to export the certificate to .pem format. This certificate can then be used on the server side of your app infrastructure to connect to the APN and send push notifications to targeted devices.
This step is pretty straight forward and described in Apple's documentation linked above.
5. Code iOS application to register for and respond to notifications
Your iOS app must register with the device (which in turns registers with the APN) to receive notifications. The best place to do this is in applicationDidFinishLaunching:, for example:
[[UIApplication sharedApplication]registerForRemoteNotificationTypes:(UIRemoteNotificationTypeBadge | UIRemoteNotificationTypeSound)];
The code above will make the device initiate a registration process with the APN. If this succeeds, the didRegisterForRemoteNotificationsWithDeviceToken: application delegate is invoked:
- (void)application:(UIApplication *)app didRegisterForRemoteNotificationsWithDeviceToken:(NSData *)devToken { const void *devTokenBytes = [devToken bytes]; self.registered = YES; [self sendProviderDeviceToken:devTokenBytes]; // custom method
Notice that the delegate is passed the deviceToken. This token is NOT the same as the UDID which is an identifier specific to the hardware. The deviceToken is specific to the instance of the iOS installation, i.e. it will migrate to a new device if the user were to restore a backup in iTunes onto a new device. The interesting thing to note here is that the deviceToken is static across apps on the specific iOS instance, i.e. the same deviceToken will be returned to other apps that register to send push notifications.
The sendProviderDeviceToken: method sends the deviceToken to the provider (i.e. your server side implementation) so the provider can use it to tell the APN which device to send the targeted push notification to.
In the case where your application is not running and the iOS device receives a remote push notification from the APN destined for your app, the didFinishLaunchingWithOptions: delegate is invoked and the message payload is passed. In this case, you would handle and process the notification in this method. If your app is already in the running, then the didReceiveRemoteNotification: method is called (you can check the applicationState property to figure out if the application is active or in the background and handle the event accordingly).
6. Implement provider communication with the APN
With the provider keys obtained in step 4, you can implement server side code to send a push notification to your users.

As illustrated in Figure 3, you will need the deviceToken of the specific user you want to send the notification to (this is the token you would have captured from the device invoking your implementation of the sendProviderDeviceToken method described in Step 4). The Identifier is an arbitrary value that identifies the notification (you can use this to correlate an error-response. Please see Apple's documentation for details). The actual payload is in JSON format.
Security Best Practices
Now that we have established details on implementing push-notifications into custom apps, let's discuss applicable security best practices along with abuse cases.
1. Do not send company confidential data or intellectual property in the message payload
Even though end points in the APN architecture are TLS encrypted, Apple is able to see your data in clear-text. There may be legal ramifications of disclosing certain types of information to third-parties such as Apple. And I bet Apple would appreciate it too (they wouldn't want the liability).
2. Push delivery is not guaranteed so don't depend on it for critical notifications
Apple clearly states that the APN service is best-effort delivery.
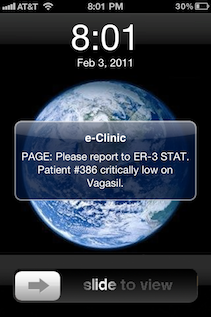
As shown in Figure 4, the push architecture should not be relied upon for critical notifications. In addition, iPhones that are not connected to cellular data (or when the phone has low to no signal) MAY not receive push notifications when the display is off for a specific period since wifi is often automatically turned off to preserve battery.
3. Do not allow the push notification handler to modify user data
The application should not delete data as a result of the application launching in response to a new notification. An example of why this is important is the situation where a push notification arrives while the device is locked: the application is immediately invoked to handle the pushed payload as soon as the user unlocks the device. In this case, the user of your app may not have intended to perform any transaction that results in the modification of his or her data.
4. Validate outgoing connections to the APN
The root Certificate Authority for Apple's certificate is Entrust. Make sure you have Entrust's root CA certificate so you are able to verify your outgoing connections (i.e. from your server side architecture) are to the legitimate APN servers and not a rogue entity.
5. Be careful with unmanaged code
Be careful with memory management and perform strict bounds checking if you are constructing the provider payload outbound to the APN using memory handling API in unmanaged programming languages (example: memcpy).
6. Do not store your SSL certificate and list of deviceTokens in your web-root
I have come across instances where organizations have inadvertently exposed their Apple signed APN certificate, associated private key, and deviceTokens in their web-root.
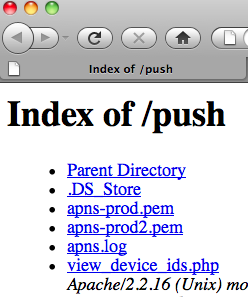
The illustration in Figure 5 is a real screen-shot of an iOS application vendor inadvertently exposing their APN certificates as well as a PHP script which lists all deviceTokens of their customers.
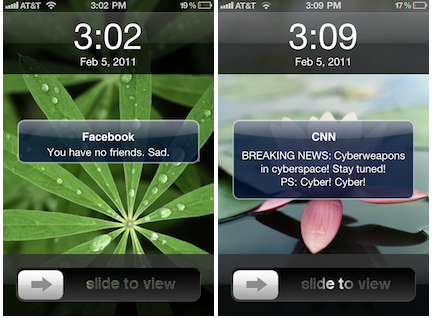
In this abuse case, any malicious entity who is able to get at the certificates and list of deviceTokens will be able to send arbitrary push notifications to this iOS application vendor's customers (see Figure 6). For example, the jpoz-apns Ruby gem can be used to send out concurrent rogue notifications:
APNS.host = 'gateway.push.apple.com' APNS.pem = '/path/to/pwn3d/pem/file' APNS.pass = " APNS.port = 2195 stolen_dtokens.each do |dtoken| APNS.send_notification(dtoken,?pwn3d') end
I would also highly recommend protecting your cert with a passphrase (it is assumed to be null in the above example). And it goes without saying that you should also keep any sample server side code that has the passphrase embedded in it out of your web-root. In fact, there is no good reason why any of this information should even reside on a host that is accessible from the Internet (incoming) since the provider connections to the APN need to be outbound only.
Conclusion
I feel Apple has done a good job of thinking through security controls to apply in the APN architecture. I hope the suggestions in this article help you to think through how to make sure the APN implementation in your app and architecture is secure from potential abuses.
If you liked this post check out SANS' new course on Secure iOS App Development.