Tags:
Guest Editor: Today's post is from Taras Kholopkin. Taras is a Solutions Architect at SoftServe, Inc. In this post, Taras will take a look at the data validation features built into the ASP.NET MVC framework.
Data validation is one of the most important aspects of web app development. Investing effort into data validation makes your applications more robust and significantly reduces potential loss of data integrity.
Out of the box, the ASP.NET MVC framework provides full support of special components and mechanisms on both the client side and the server side.
Client-Side Validation
Enabled Unobtrusive JavaScript validation allows ASP.NET MVC HTML helper extensions to generate special markup to perform validation on the client side, before sending data to the server. The feature is controlled by the "UnobtrusiveJavaScriptEnabled" Boolean setting in the section.
Let's have a look at the Register page from the SecureWebApp project in the previous article.
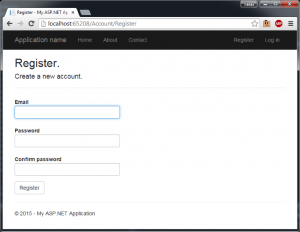
The Register.cshtml view contains the following code declaring the text box field for entering a user's email:
@model SecureWebApp.Models.RegisterViewModel ... @Html.TextBoxFor(m => m.Email, new { @class = "form-control" })
With unobtrusive JavaScript validation enabled, the framework transforms this code into the following HTML markup:
<input class="form-control valid" data-val="true" data-val-email="The Email field is not a valid e-mail address." data-val-required="The Email field is required." id="Email" name="Email" type="text" value="" >
You can see the validation attributes, data-val, data-val-email, and data-val-required, generated by the framework. Entering an invalid email address, the client-side validation executes and displays the error message to the user. More information about input extensions and available methods can be found here.
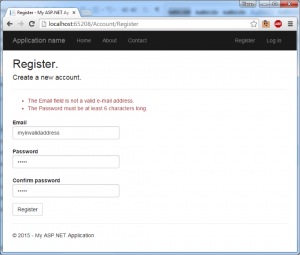
Server-side Validation
While client-side data validation is user friendly, it is important to realize that it does not provide any security for our application. Browsers can be manipulated and web application proxies can be used to bypass all client-side validation protections.
For this reason, performing server-side validation is critical to the application's security. The first step is using the data model validation annotations in the System.ComponentModel.DataAnnotations namespace, which provides a set of validation attributes that can be applied declaratively to the data model. Looking at the RegisterViewModel class, observe the attributes on each property. For example, the Email property uses the [Required] and [Email] annotations, which tell the server a value must be entered by the user and that value must be a valid email address.
public class RegisterViewModel { [Required] [EmailAddress] [Display(Name = "Email")] public string Email { get; set; } [Required] [StringLength(100, ErrorMessage = "The {0} must be at least {2} characters long." , MinimumLength = 6)] [DataType(DataType.Password)] [Display(Name = "Password")] public string Password { get; set; }
Next, the server-side action that processes the model must check the ModelState.IsValid property before accepting the request. The following example shows the AccountController Register method checking if the model is valid before registering the user's account.
[HttpPost] [AllowAnonymous] [ValidateAntiForgeryToken] public async Task Register(RegisterViewModel model) { if (ModelState.IsValid) { var user = new ApplicationUser { UserName = model.Email, Email = model.Email }; var result = await UserManager.CreateAsync(user, model.Password); if (result.Succeeded) { await SignInManager.SignInAsync(user, isPersistent:false, rememberBrowser:false); return RedirectToAction("Index", "Home"); } AddErrors(result); } // If we got this far, something failed, redisplay form return View(model); }
To demonstrate server-side validation in action, let's disable "UnobtrusiveJavaScriptEnabled" option in web.config and try to register a user with an invalid email address and password. Using the ModelState.IsValid check, the server-side validation results in the same error message as we had with client-side validation enabled.
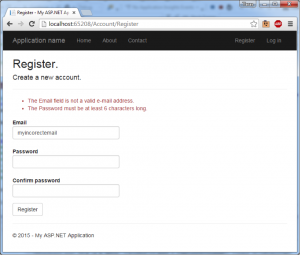
ASP.NET provides several built-in validation annotations that can be used in your applications including file extensions, credit card numbers, max length, min length, phone number, numeric ranges, URL, and regular expressions. Or, you can use the ValidationAttribute class to create a custom annotation for your model classes. We will explore creating custom validation attributes in a future post.
To learn more about securing your .NET applications with ASP.NET MVC validation, sign up for DEV544: Secure Coding in .NET!
Taras Kholopkin is a Solutions Architect at SoftServe, and a frequent contributor to the SoftServe United blog. Taras has worked more than nine years in the industry, including extensive experience within the US IT Market. He is responsible for software architectural design and development of Enterprise and SaaS Solutions (including Healthcare Solutions).