Tags:
Backgrounding and Snapshots
In iOS when an application moves to the background the system takes a screen shot of the application's main window. This screen shot is used to animate transitions when the app is reopened. For example, pressing the home button while using the logon screen of the Chase App results in the following screen shot being saved to the application's Library/Caches/Snapshots/com.chase directory.
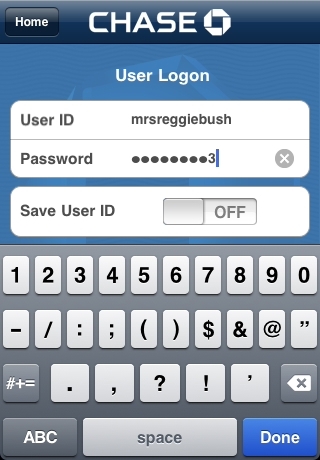
Example Application
To further illustrate this point take the following profile page from a fictitious bank app which displays sensitive information like the user's account number, balance, and secret question/answer.
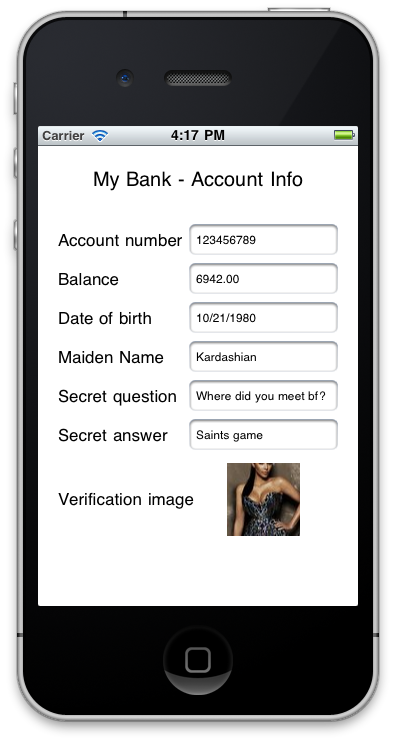
If the user presses the home button while viewing this screen a snapshot of the window will be saved to the application's Snapshots directory. If you run this code in the iOS Simulator the snapshot is stored in the ~/Library/Application Support/iPhone Simulator/4.2/Applications//Library/Caches/Snapshots/com.yourcompany.MyBank directory.
Hiding Sensitive Data
The iOS Application Programming Guide states that sensitive information should be removed from views before moving to the background. Specifically, it states that when "the applicationDidEnterBackground: method returns, the system takes a picture of your application's user interface...If any views in your interface contain sensitive information, you should hide or modify those views before the applicationDidEnterBackground: method returns."
Fortunately, the code for hiding the sensitive fields in the fictitious "My Bank" application is very straightforward. In the delegate you can simply mark the sensitive fields as hidden:
- (void)applicationDidEnterBackground:(UIApplication *)application { viewController.accountNumberField.hidden = YES; viewController.balanceField.hidden = YES; viewController.dobField.hidden = YES; viewController.maidenNameField.hidden = YES; viewController.secretQuestionField.hidden = YES; viewController.secretAnswerField.hidden = YES; }
Of course, you also need to make the fields visible before the app becomes active using the following code in applicationDidBecomeActive:
- (void)applicationDidBecomeActive:(UIApplication *)application { viewController.accountNumberField.hidden = NO; viewController.balanceField.hidden = NO; viewController.dobField.hidden = NO; viewController.maidenNameField.hidden = NO; viewController.secretQuestionField.hidden = NO; viewController.secretAnswerField.hidden = NO; }
Adding this code to the delegate results in the following screen shot (without sensitive data) being taken when the home button is pressed.
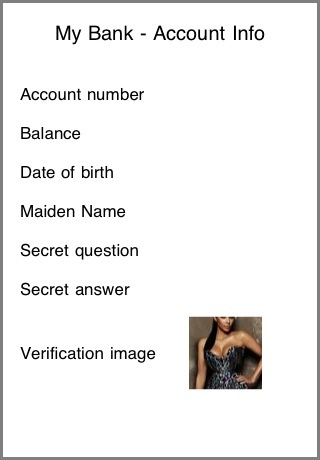
Preventing Backgrounding
Instead of hiding or removing sensitive data you can also prevent backgrounding altogether by setting the "Application does not run in background" property in the application's Info.plist file (this adds the UIApplicationExitsOnSuspend key to the plist). Setting this property results in applicationWillTerminate: being called and prevents the screenshot from being taken at all.
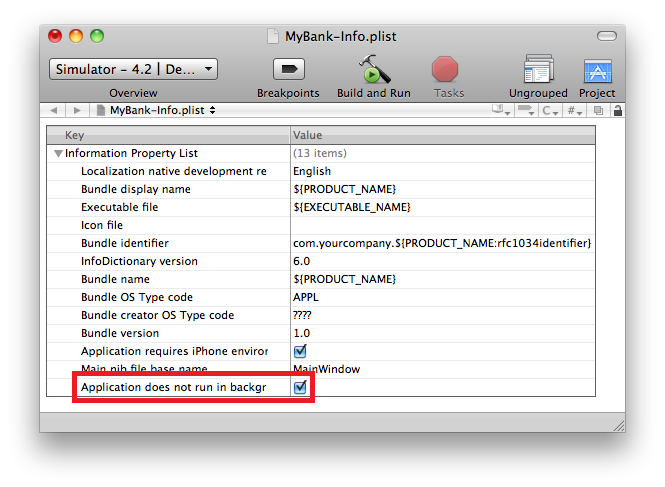
Summary
Sensitive data can be inadvertently saved when an app moves to the background. Developers should mitigate this by identifying sensitive fields and implementing applicationDidEnterBackground: or by preventing backgrounding altogether.